Before you begin: Join our book community on Discord
Give your feedback straight to the author himself and chat to other early readers on our Discord server (find the “architecting-aspnet-core-apps-3e” channel under EARLY ACCESS SUBSCRIPTION).
https://packt.link/EarlyAccess
This chapter explores two new design patterns from the well-known Gang of Four (GoF). They are behavioral patterns, meaning they help simplify system behavior management.Often, we need to encapsulate some core algorithm while allowing other pieces of code to extend that implementation. That is where the Template Method pattern comes into play.Other times, we have a complex process with multiple algorithms that all apply to one or more situations, and we need to organize it in a testable and extensible fashion. This is where the Chain of Responsibility pattern can help. For example, the ASP.NET Core middleware pipeline is a Chain of Responsibility where all the middleware inspects and acts on the request.In this chapter, we cover the following topics:
- Implementing the Template Method pattern
- Implementing the Chain of Responsibility pattern
- Mixing the Template Method and Chain of Responsibility patterns
Implementing the Template Method pattern
The Template Method is a GoF behavioral pattern that uses inheritance to share code between the base class and its subclasses. It is a very powerful yet simple design pattern.
Goal
The goal of the Template Method pattern is to encapsulate the outline of an algorithm in a base class while leaving some parts of that algorithm open for modification by the subclasses, which adds flexibility at a low cost.
Design
First, we need to define a base class that contains the TemplateMethod method and then define one or more sub-operations that need to be implemented by its subclasses (abstract) or that can be overridden (virtual).Using UML, it looks like this:
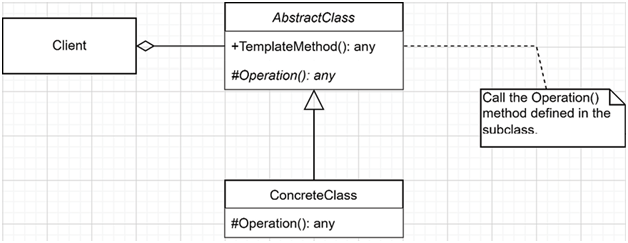
Figure 12.1: Class diagram representing the Template Method pattern
How does this work?
- AbstractClass implements the shared code: the algorithm in its TemplateMethod method.
- ConcreteClass implements its specific part of the algorithm in its inherited Operation method.
- Client calls TemplateMethod(), which calls the subclass implementation of one or more specific algorithm elements (the Operation method in this case).
We could also extract an interface from AbstractClass to allow even more flexibility, but that’s beyond the scope of the Template Method pattern.
Let’s now get into some code to see the Template Method pattern in action.